Preview Search API
Overview
The Preview Search API supports our Person Search API by providing a preview of which fields have data in each record matching a search query.
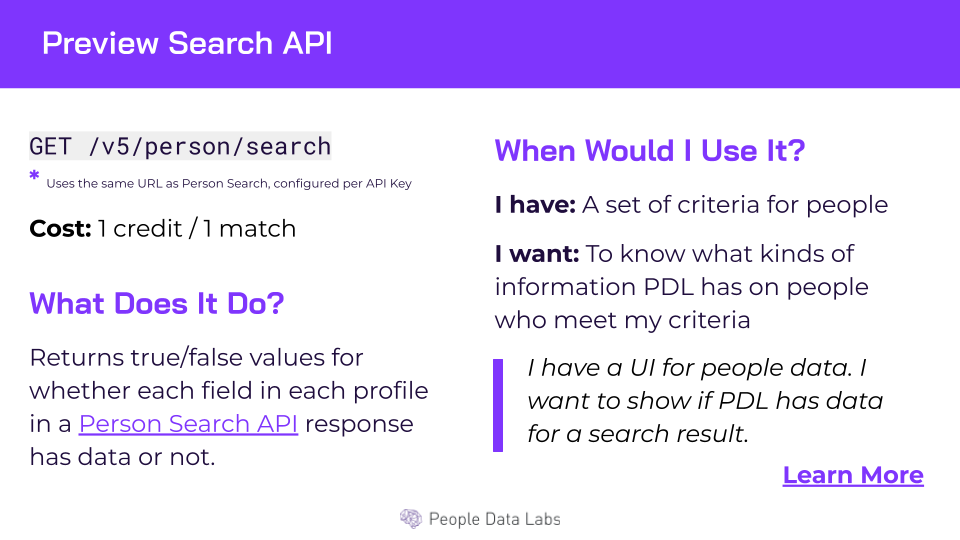
Request Format
The endpoint for the Preview Search API is https://api.peopledatalabs.com/v5/person/search
.
Creating an API Key for Preview Search
The Preview Search API uses the same endpoint as the Person Search API. Your API Key will determine whether you use the Person Search or Preview Search API.
To create an API Key that supports the Person Search Preview API, speak with your data consultant.
See Input Parameters - Person Search API for details on the supported parameters. All valid input parameters for the Person Search API will work the same way for the Preview Search API.
Example
import requests, json
# Set your API key
API_KEY = "YOUR PREVIEW SEARCH ENABLED API KEY"
# Set the Preview Search API URL
PDL_URL = "https://api.peopledatalabs.com/v5/person/search"
# Set the headers
HEADERS = {
"X-Api-Key": API_KEY
}
# Create an Elasticsearch query
ES_QUERY = {
"query": {
"bool": {
"must": [
{"term": {"job_company_name": "people data labs"}}
]
}
}
}
# Create a parameters JSON object
PARAMS = {
'query': json.dumps(ES_QUERY),
'size': 10,
'pretty': True
}
# Pass the parameters object to the Person Enrichment API
json_response = requests.get(PDL_URL, headers=HEADERS, params=PARAMS).json()
# Check for successful response
if json_response["status"] == 200:
record = json_response['data']
# Save preview data to JSON file
with open("my_pdl_search_preview.jsonl", "w") as out:
out.write(json.dumps(record) + "\n")
else:
print("Search preview unsuccessful. See error and try again.")
print("error:", json_response)
import requests, json
# Set your API key
API_KEY = "YOUR PREVIEW SEARCH ENABLED API KEY"
# Set the Preview Search API URL
PDL_URL = "https://api.peopledatalabs.com/v5/person/search"
# Set the headers
HEADERS = {
"X-Api-Key": API_KEY
}
# Create a SQL query
SQL_QUERY = \
"""
SELECT * FROM person
WHERE job_company_name='people data labs';
"""
# Create a parameters JSON object
PARAMS = {
'query': SQL_QUERY,
'size': 10,
'pretty': True
}
# Pass the parameters object to the Person Enrichment API
json_response = requests.get(PDL_URL, headers=HEADERS, params=PARAMS).json()
# Check for successful response
if json_response["status"] == 200:
record = json_response['data']
# Save preview data to JSON file
with open("my_pdl_search_preview.jsonl", "w") as out:
out.write(json.dumps(record) + "\n")
else:
print("Search preview unsuccessful. See error and try again.")
print("error:", json_response)
# Elasticsearch
curl -X GET 'https://api.peopledatalabs.com/v5/person/search' \
-H 'X-Api-Key: xxxx' \
--data-raw '{
"size": 10,
"query": {
"bool": {
"must": [
{"term": {"job_company_name": "people data labs"}}
]
}
}
}'
# SQL
curl -X GET \
'https://api.peopledatalabs.com/v5/person/search' \
-H 'X-Api-Key: xxxx' \
--data-raw '{
"size": 10,
"sql": "SELECT * FROM person WHERE job_company_name='\''people data labs'\'';"
}'
Response Format
The Preview Search API is a wrapper on the Person Search API, meaning it returns all of the same fields as the Person Search API, but with true / false values instead (true meaning we have a value for that field and false meaning we do not have a value for it).
Any nested objects will not be included in the Preview Search response. For example, if a record has data for the education
field, the response will have "education": true
, but will not list any of the subfields (such as education.major
).
To help users know which record they're looking at, the following fields will have the actual data if it exists for the record: id
, full_name
, sex
, linkedin_url
, industry
, job_title
, job_title_levels
, job_company_name
, job_company_website
, location_name
. All other fields will have true/false values as described above.
The output is formatted as such:
Field Name | Type | Description |
---|---|---|
status | Integer | The HTTP status code. |
data | Array (Object) | The person response objects that match the input query. |
scroll_token | String | The scroll token to use to fetch the next batch of results for the query. |
total | Integer | The total number of search results matching the input query. |
Full Example Response
{
"status": 200,
"data": [
{
"id": "qEnOZ5Oh0poWnQ1luFBfVw_0000",
"full_name": "sean thorne",
"sex": "male",
"linkedin_url": "linkedin.com/in/seanthorne",
"industry": "computer software",
"job_title": "co-founder and chief executive officer",
"job_title_role": null,
"job_title_sub_role": null,
"job_title_levels": [
"owner",
"cxo"
],
"job_company_name": "people data labs",
"job_company_website": "peopledatalabs.com",
"location_name": "san francisco, california, united states",
"birth_date": false,
"birth_year": true,
"countries": true,
"education": true,
"emails": true,
"experience": true,
"facebook_id": true,
"facebook_url": true,
"facebook_username": true,
"first_name": true,
"github_url": false,
"github_username": false,
"interests": true,
"job_company_facebook_url": true,
"job_company_founded": true,
"job_company_id": true,
"job_company_industry": true,
"job_company_linkedin_id": true,
"job_company_linkedin_url": true,
"job_company_location_address_line_2": true,
"job_company_location_continent": true,
"job_company_location_country": true,
"job_company_location_geo": true,
"job_company_location_locality": true,
"job_company_location_metro": true,
"job_company_location_name": true,
"job_company_location_postal_code": true,
"job_company_location_region": true,
"job_company_location_street_address": true,
"job_company_size": true,
"job_company_twitter_url": true,
"job_last_updated": true,
"job_last_changed": true,
"job_last_verified": true,
"job_start_date": true,
"last_initial": true,
"last_name": true,
"linkedin_id": true,
"linkedin_username": true,
"location_address_line_2": false,
"location_continent": true,
"location_country": true,
"location_geo": true,
"location_last_updated": true,
"location_locality": true,
"location_metro": true,
"location_names": true,
"location_postal_code": false,
"location_region": true,
"location_street_address": false,
"middle_initial": true,
"middle_name": true,
"mobile_phone": true,
"personal_emails": false,
"phone_numbers": true,
"profiles": true,
"regions": true,
"skills": true,
"street_addresses": false,
"twitter_url": true,
"twitter_username": true,
"work_email": true
},
...
],
"scroll_token": "1117$12.176522",
"total": 10
}
Field Availability
The Preview Search API will only return the fields shown in the example response above. Even if you have purchased additional fields or bundles for Person Search, those fields will not show in the Preview Search response.
Errors
If the request encounters an error, it will instead return an Error Response in the format described in Errors.
Access & Billing
The Preview Search API is available for purchase by enterprise API customers. If you’d like access, please reach out to us.
The Preview Search API uses its own set of credits. To add Preview Search credits to your Preview Search API Key, speak with your data consultant.
Updated 6 months ago